SpringAI:最好的 Java AI编程框架
Spring AI 是最好的 Java AI 编程框架,其目标是将 Spring 生态系统的可移植性和模块化设计等设计原则应用于 AI 编程领域,并且方便的将企业数据/企业API和AI模型连接起来。
Spring AI 集成 Ollama 本地模型
工程搭建
在 SpringBoot 脚手架页面 按照下图所示进行参数选择,之后点击“GENERATE”生成代码包。
解压该包,引入 IDEA。
代码编写
引入统一依赖管理 bom 配置
xml
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.ai</groupId>
<artifactId>spring-ai-bom</artifactId>
<version>1.0.0-M8</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
引入 ollama 模型自动装配 starter
xml
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<!-- web -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- model -->
<dependency>
<groupId>org.springframework.ai</groupId>
<artifactId>spring-ai-starter-model-ollama</artifactId>
</dependency>
</dependencies>
application.properties 配置文件
spring.ai.ollama.base-url=http://127.0.0.1:11434
spring.ai.ollama.chat.options.model=qwen3:32b
说明:列出最核心的两个配置,更多配置项见 spring-ai-ollama 文档
- spring.ai.ollama.base-url:ollama 服务的基础 url
- spring.ai.ollama.chat.options.model:指定使用的 ollama 服务
ChatClient Bean 配置
java
@Configuration
public class SpringAIConfig {
@Bean
public ChatClient chatClient(ChatModel chatModel) {
return ChatClient.create(chatModel);
}
}
controller:
java
@RequestMapping("/chat")
@RestController
public class SpringAiChatController {
@Resource
private ChatClient chatClient;
@RequestMapping("/ollama")
public String ollama() {
return chatClient
.prompt()
.user("今天是几月几日,苏州天气怎么样")
.call().content();
}
}
启动器:
java
@SpringBootApplication
public class SpringAiApplication {
public static void main(String[] args) {
SpringApplication.run(SpringAiApplication.class, args);
}
}
启动应用
启动应用,浏览器访问 http://localhost:8080/chat/ollama
,查看输出即可
text
好的,用户问今天几月几日和苏州的天气。首先,我需要确认今天的日期。但作为AI,我无法实时获取当前日期,所以需要提醒用户这一点。然后是关于苏州的天气,同样需要实时数据,比如温度、天气状况、是否有雨等。用户可能计划外出或安排活动,所以天气信息对他们来说很重要。我应该建议用户查看可靠的天气预报网站或应用,比如中国天气网或AccuWeather。
从输出可以看出 Qwen3 模型本身无法获取本地时间和天气,此时可以用使用 mcp 能力解决。
Spring AI 集成 MCP 服务
引入 mcp-server 依赖
xml
<!-- mcp-server -->
<dependency>
<groupId>org.springframework.ai</groupId>
<artifactId>spring-ai-starter-mcp-server</artifactId>
</dependency>
编写两个 mcp 服务
java
@Service
public class TimeMCP {
@Tool(description = "获取当前系统的时间")
public String getSystemTime() {
return "2025-05-05";
}
}
@Service
public class WeatherMCP {
@Tool(description = "获取指定城市的天气")
public String getWeather(String cityName) {
if ("杭州".equals(cityName)) {
return "21度";
}
return "22度";
}
}
注册 mcp 服务
java
@Bean
public ToolCallbackProvider weatherTools(WeatherMCP weatherMCP) {
return MethodToolCallbackProvider.builder().toolObjects(weatherMCP).build();
}
@Bean
public ToolCallbackProvider timeTools(TimeMCP timeMCP) {
return MethodToolCallbackProvider.builder().toolObjects(timeMCP).build();
}
模型自动调用 mcp 服务
java
@RequestMapping("/chat")
@RestController
public class SpringAiChatController {
@Resource
private ChatClient chatClient;
@Resource
private ToolCallbackProvider weatherTools;
@Resource
private ToolCallbackProvider timeTools;
@RequestMapping("/ollama")
public String ollama() {
return chatClient
.prompt()
.user("今天是几月几日,苏州天气怎么样")
.toolCallbacks(weatherTools, timeTools) // 注明可用 mcp-server 列表
.call().content();
}
}
启动服务后,访问 http://localhost:8080/chat/ollama
,查看输出如下:
text
好的,用户问今天的日期和苏州的天气。
首先,我需要调用getSystemTime函数来获取当前系统时间。这个函数不需要参数,所以直接调用。
然后,再调用getWeather函数,参数是苏州。
接下来,处理返回的日期是2025年5月5日,天气是22度。
需要把这些信息用自然的中文组织起来,告诉用户今天是5月5日,苏州的天气情况。要注意日期格式转换,把"2025-05-05"转换成“5月5日”,温度直接显示22度。
最后确保回答简洁明了,符合用户的需求。 今天是2025年5月5日,苏州的天气是22度。
文章的最后,如果您觉得本文对您有用,请打赏一杯咖啡!感谢!
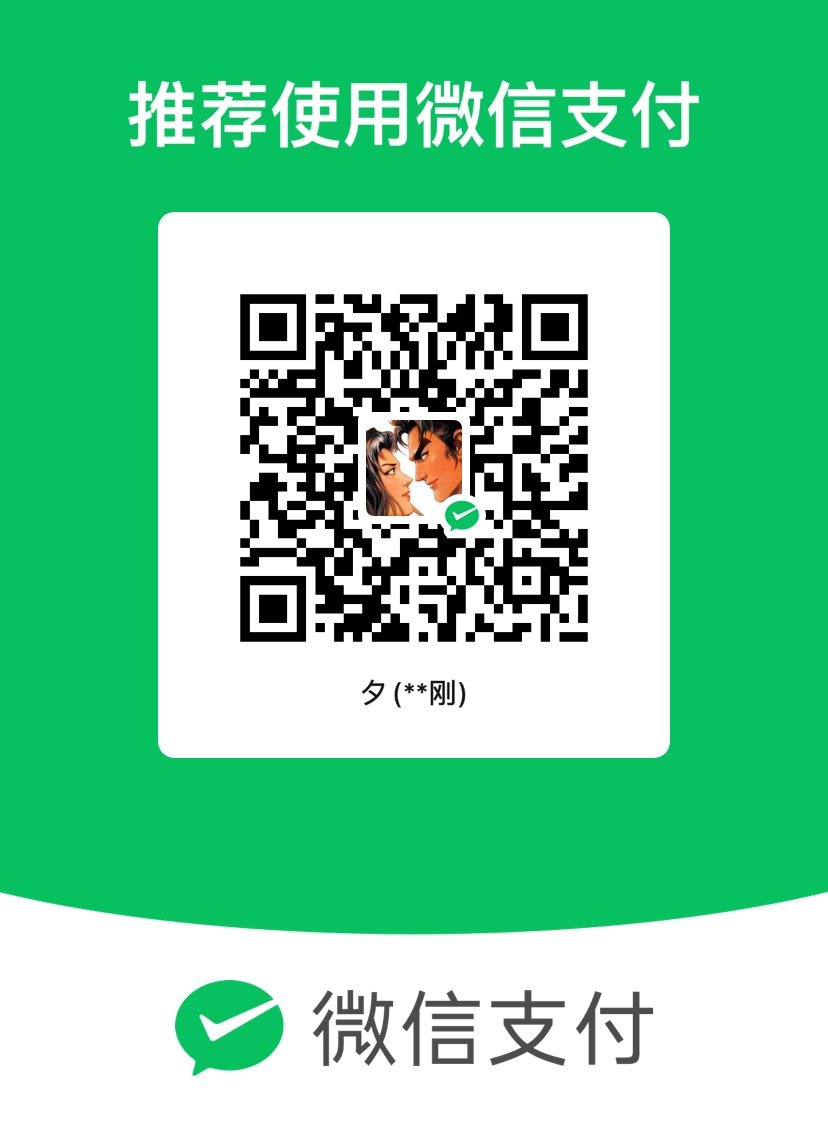